But before we talk about it, we need to solve a problem with our input function that receives data from the user's keyboard.
input() Function and returning strings
When we type anything on the keyboard for the capture input, it will automatically transform that data into a string (a text).Warning: This is in the newer version of Python, the 3.x (3.4, 3.6 ... etc).
UPDATE YOUR PYTHON FOR THE NEWER VERSION, OK?
Let's use the following script that asks for three user data, and then displays the type of variable through the type() function.
Our script:
string=input("Type a string: ") print( type(string) ) integer=input("Type a integer: ") print( type(integer) ) decimal=input("Type a float: ") print( type(decimal) )
Now let's run and type a string, then an integer and then a float (decimal), see the result:
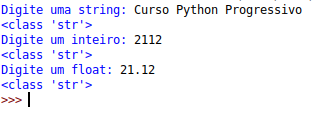
Note that in all three cases, the variables are of type string ('str').
But, Python!
I wanted only the first string!
2112 wanted it to be an integer
21.12 wanted to be a decimal (float)
Why does it happen? Did you bug in Python? Did I break Python?
No, dear paladin of the computational arts.
This is a feature of the input() function in Python 3.x
We say that the input function returns a string.
That is, when we use it, it will put a string in the variable, no matter what you typed, okay?
But let's learn how to turn those unwanted strings into numbers of any kind!
int() Function - String to integer (str to int) in Python
To convert a string to integer, let's use the int() function.Just put whatever we want between the parentheses of this int(), which returns an integer.
In case, let's put in a string, okay?
Examples:
Let's define a variable called var1 and put the string '2112' inside it.
Next, let's use the type() function to display the data type ('string' will appear).
Then we get another var2 variable and get it to get the int() function and inside that int, we put the string var1. Then we print the data type that is var2, see:
var1='2112' print( type(var1) ) var2 = int(var1) print( type(var2) )
As we expected, the result
We transformed the string '2112' to the number 2112 !
float() Function- String to Decimal (str to float) in Python
Exactly how int() works, float() works.Everything we put between the parentheses of this function, it will transform into float.
Let's create a script that asks for a decimal for the user and stores it in variable var1. Then we print the datatype of this var1, which will be 'str'.
We then place this var1 inside the float() function and store the value in a variable var2. Next, we print the data type of this variable, which will be 'float'.
Now, let's get a variable var3 and put an integer in it, the number 2112, which is the most fucking number of all.
Then we transform this integer into a float, store it in var4 and see the data type of var4, which is now float, see:
var1=input("Enter a decimal: ") print( type(var1) ) var2=float(var1) print( type(var2) ) var3=2112 var4=float(var3) print( type(var4) ) print(var4)
The result:
Nice! We transform a string into float, and then the integer 2112 into a float. We printed up var4 to see that 2112 became 2112.0, a decimal!
Using int() e float() in input() function
Okay, but what about our initial problem? We want a number and the damn of the input function give me a string, how to solve it?Very simple. Remember that we said that the input returns a string?
So just play the input function inside the functions int() and float()
That is, to take a user data, transform into integer and store in variable var1, do:
var1 = int( input("Type a integer: ") )
And to get the user's data and turn it into decimal, just play the input inside the float function:
var2 = float( input("Type a decimal: ") )
And ready! var1 is an integer data type and var2 is a float type.
Simple, right?
No comments:
Post a Comment