We proposed that you create a calculator in Python, which asks the user for two numbers and displays all operations as follows:
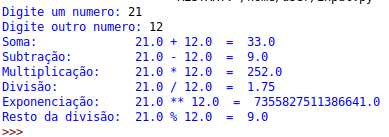
Now let's show the solution to this exercise in two ways.
But first, we need to learn something essential in programming: commenting on a code.
How to comment a code - Char: #
One of the most essential things you need to learn in programming is commenting your code."There goes Python, it starts executing. Python read variable, calls input, input passes to int function that crosses to print function and displays result on screen, it's goal!"
No, nothing to see, you will not comment or narrate anything.
Comment is to write things in your code, in your language (Portuguese, English ...) so that whoever is going to see the code, can read something.
Let's go back in time and type Hello, World.
Write and run:
# The program below displays a message on the screen print("Hello, World!")
Notice that there is something different. On the first line.
We write the game symbol hash and write something.
However, when we run our script, this line does not appear.
That's what a comment is: a line that starts with the #
Python simply ignores everything starting with #
You can put anything, any command, up to a letter for Trump after # that Python will not read, will not rotate that line nor will anything appear in the interpreter.
Now it may seem silly to use this, but as your programs get huge, complex, and difficult, it's highly recommended that you go over your code, type
# Here we are connecting with the NASA website
[code]
# Now let's try to invade the NASA system
[code]
# Having access to the NASA database
[code]
# The following code begins downloading NASA's secret files
[code]
# Oh damn! They got me
This helps you when you see the code again, to understand what each part of the code is doing, what good it is. OK ?
Always comment your codes!
Let's give an example of commented code now, making the calculator in Python.
Simple Calculator in Python
The code of our calculator, all cute, organized and commented like this:# Initially, we asked for the two numbers for the user # Let's turn it into float var1 = float( input("Type a number: ") ) var2 = float( input("Enter another: ") ) # Calculating the sum and storing in the variable 'sum' sum = var1 + var2 # Calculating the subtraction and storing in the variable 'sub' subt = var1 - var2 # Calculating multiplication and storing in variable 'mult' mult = var1 * var2 # Calculating the division and storing in the variable 'div' div = var1 / var2 # Calculating exponentiation and storing in variable 'expo' exp = var1 ** var2 # Calculating the remainder of the division and storing in the variable 'resto' remainder = var1 % var2 # Imprimindo tudo print('Sum: ', var1,'+',var2,' = ' , sum) print('Sub: ', var1,'-',var2,' = ' , sub) print('Multiplying: ', var1,'*',var2,' = ' , mult) print('Division ', var1,'/',var2,' = ' , div) print('Exponentiation: ', var1,'**',var2,' = ', exp) print('Remainder: ', var1,'%',var2,' = ' , remainder)
And yours?
Put your code in the comments.
Smaller Calculator Code in Python
One of the most common things to do with whoever is starting to program is to create long, messy, confusing codes.Sometimes you program take 200 lines of code to do something.
HTHEN,re comes a son of a... and makes a better, error-free, more organized code that runs faster and using only 30 lines.
It gives a rage ... but, calm down. That's just the beginning.
The following code is the same as the calculator, but it is smaller, runs faster and consumes less memory because we use only two variables 'var1' and 'var2':
# Receiving data from uservar1 = float( input("Type a number: ") ) var2 = float( input("Enter another: ") )# Print the result of operations directly on the print function print('Sum: ', var1,'+',var2,' = ' , var1+var2) print('Sub: ', var1,'-',var2,' = ' , var1-var2) print('Multiplication: ', var1,'*',var2,' = ' , var1*var2) print('Division: ', var1,'/',var2,' = ' , var1/var2) print('Exponentiation: ', var1,'**',var2,' = ', var1**var2) print('Remainder: ', var1,'%',var2,' = ' , var1%var2)
Nice, is not it?